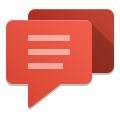
![]() |
MoYi123V2EX 第 469223 号会员,加入于 2020-02-14 14:02:50 +08:00 |
有没有一种通过 git flow 判断公司或者团队靠谱程度的办法 奇思妙想 • MoYi123 • 2020-06-19 18:30:11 PM • 最后回复来自 libook | 8 |
7 天前 回复了 chenxiaolani 创建的主题 › 程序员 › 后端接口一定要保持单一职责吗 |
7 天前 回复了 shrugginG 创建的主题 › MySQL › mysql 小白请教大佬一个问题 |
9 天前 回复了 iqoo 创建的主题 › 程序员 › 分享一个空间利用率超高的 Base36 算法 |
11 天前 回复了 szpinc1102 创建的主题 › 程序员 › 网上看到的段子照进了现实,这种代码出现在我的项目中! |
12 天前 回复了 huangsijun17 创建的主题 › Linux › [求助] 在百万到千万级个文件中查找最大的几个。 |
12 天前 回复了 V392920 创建的主题 › 程序员 › 大佬们,来寻求个方案,对比查询怎么才能最快 |
13 天前 回复了 gotoschool 创建的主题 › 生活 › 大家说说自己的日生活成本 |
13 天前 回复了 name1991 创建的主题 › 职场话题 › 现在出来面试都要写算法题吗? |